Empower Your Security Posture: AI Chatbots - Detect, Analyze, Protect!
- Bhola Suryavanshi
- Jan 28
- 2 min read
Updated: Feb 9
A detailed explanation on how chatbot can be leveraged to address automate Threat Detection and Performance Analysis...
Developing a chatbot to address threat detection and perform analysis involves integrating AI and machine learning capabilities with cybersecurity tools and data sources. The chatbot acts as an interface that can process and analyze vast amounts of security-related data to identify potential threats and provide insights.
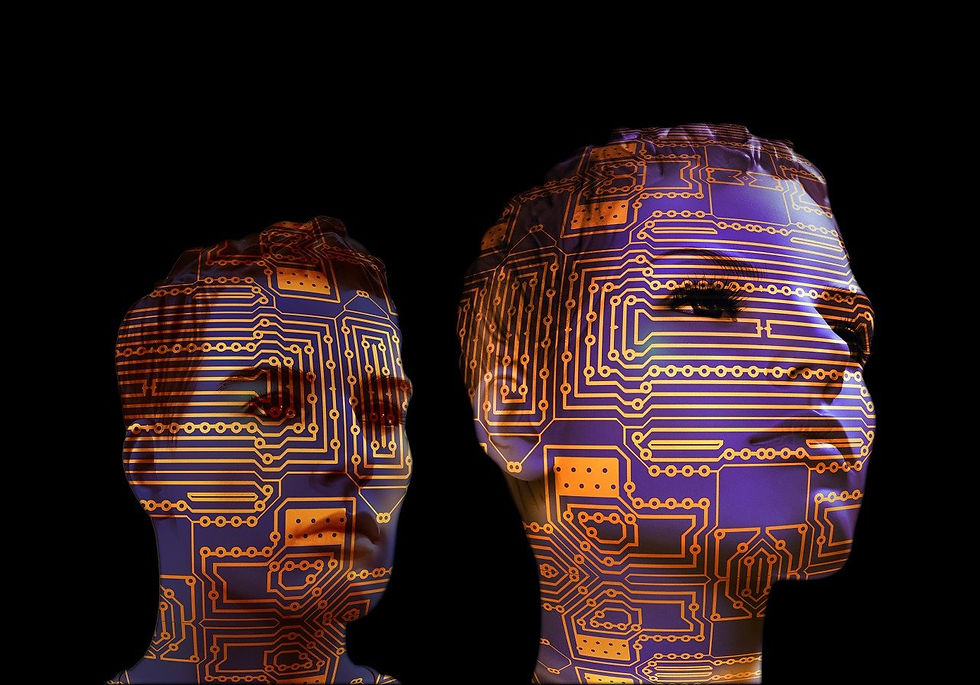
Here's an outline of the steps and components involved in developing such a chatbot:
Creating a fully functional chatbot for threat detection and analysis is a complex task that involves multiple components, including data collection, machine learning, natural language processing, and integration with existing security systems. This process requires a significant amount of custom development and is beyond the scope of a simple code snippet.
However, below is the pseudocode outline that describes the process of developing such a chatbot.
Pseudocode (Python) for a cybersecurity chatbot for threat detection and analysis
# Import necessary libraries and modules
import machine_learning_library
import natural_language_processing_library
import data_integration_library
import incident_management_system_library
# Initialize chatbot with NLP and machine learning capabilities
chatbot = initialize_chatbot(nlp_model, ml_model)
# Function to collect and integrate data from various sources
def collect_data():
network_logs = data_integration_library.collect_network_logs()
system_logs = data_integration_library.collect_system_logs()
application_logs = data_integration_library.collect_application_logs()
threat_intelligence = data_integration_library.collect_threat_intelligence()
return network_logs, system_logs, application_logs, threat_intelligence
# Function to train machine learning model for threat detection
def train_threat_detection_model(data):
model = machine_learning_library.create_model()
model.train(data)
return model
# Function to detect threats based on the analysis of collected data
def detect_threats(data, model):
threats = model.analyze(data)
return threats
# Function to prioritize detected threats
def prioritize_threats(threats):
prioritized_threats = sort_threats_by_severity(threats)
return prioritized_threats
# Function to interact with users and respond to queries
def interact_with_user(user_query):
response = chatbot.respond_to_query(user_query)
return response
# Function to guide users through incident response
def incident_response_guidance(threat):
response_procedure = incident_management_system_library.get_response_procedure(threat)
return response_procedure
# Main loop for the chatbot operation
while True:
# Collect and integrate data from sources
data = collect_data()
# Detect threats using the trained model
threats = detect_threats(data, trained_model)
# Prioritize detected threats
prioritized_threats = prioritize_threats(threats)
# Interact with users and provide analysis and guidance
user_query = get_user_query()
if user_query:
response = interact_with_user(user_query)
display_response(response)
# If a threat is confirmed, provide incident response guidance
if threat_confirmed(prioritized_threats):
guidance = incident_response_guidance(prioritized_threats[0])
display_guidance(guidance)
This pseudocode is a simplified representation of the components and logic that might be involved in creating a cybersecurity chatbot. The actual implementation would require selecting specific libraries and tools, writing detailed code for each function, training machine learning models with real data, and integrating the chatbot with existing cybersecurity infrastructure.
コメント